I have another new toy to distract me from all the things I should be doing!
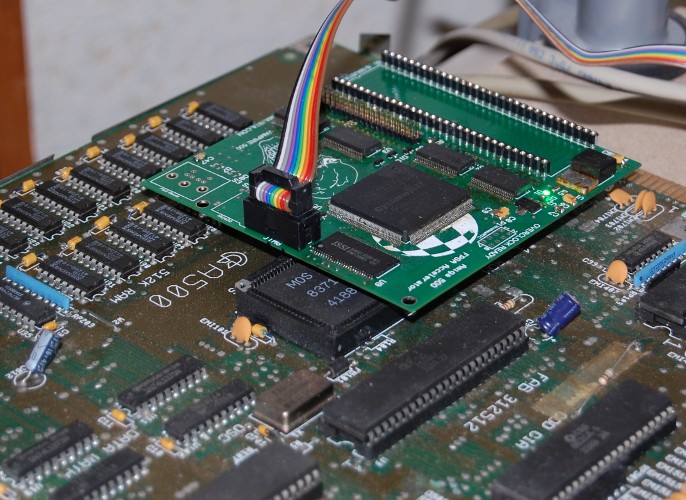
This is one of Majsta’s hand-assembled prototypes of his FPGA accelerator, connected to a spare A500 motherboard that I just happened to have lying around. (I don’t currently have an A600 to play with, which is why Majsta sent me the less-mature A500 variant of the project). While I’ve been trying to help with the SDRAM problems his project’s run into, there’s only so much I can do without access to the hardware. Well, now I have that access!
The A500 variant of the project’s not yet as complete as the A600 version, and can’t yet boot from the TG68 processor, so now begins the fun of testing the various kinds of accesses to the A500 motherboard, and ironing out the glitches.
Because the whole project takes some time to build, while I’m testing and diagnosing I’m using a simple state machine to simulate the CPU, which looks like this:
library ieee;
use ieee.std_logic_1164.all;
use ieee.std_logic_unsigned.all;
use ieee.numeric_std.all;
entity DummyCPU is
generic(
SR_Read : integer:= 0; --0=>user, 1=>privileged, 2=>switchable with CPU(0)
VBR_Stackframe : integer:= 0; --0=>no, 1=>yes/extended, 2=>switchable with CPU(0)
extAddr_Mode : integer:= 0; --0=>no, 1=>yes, 2=>switchable with CPU(1)
MUL_Mode : integer := 0; --0=>16Bit, 1=>32Bit, 2=>switchable with CPU(1), 3=>no MUL,
DIV_Mode : integer := 0; --0=>16Bit, 1=>32Bit, 2=>switchable with CPU(1), 3=>no DIV,
BitField : integer := 0 --0=>no, 1=>yes, 2=>switchable with CPU(1)
);
port(clk : in std_logic;
nReset : in std_logic; --low active
clkena_in : in std_logic:='1';
data_in : in std_logic_vector(15 downto 0);
IPL : in std_logic_vector(2 downto 0):="111";
IPL_autovector : in std_logic:='0';
CPU : in std_logic_vector(1 downto 0):="00"; -- 00->68000 01->68010 11->68020(only some parts - yet)
addr : buffer std_logic_vector(31 downto 0);
data_write : out std_logic_vector(15 downto 0);
nWr : out std_logic;
nUDS, nLDS : out std_logic;
busstate : out std_logic_vector(1 downto 0); -- 00-> fetch code 10->read data 11->write data 01->no memaccess
nResetOut : out std_logic;
FC : out std_logic_vector(2 downto 0);
-- for debug
skipFetch : out std_logic;
regin : buffer std_logic_vector(31 downto 0)
);
end DummyCPU;
architecture rtl of DummyCPU is
type states is (write1,write2,write3,write4,write5,write6,read1,read2);
signal state : states := read1;
signal counter : unsigned(15 downto 0);
signal temp : std_logic_vector(15 downto 0);
begin
process(clk)
begin
nResetOut<=nReset;
if nReset='0' then
state<=read1;
elsif rising_edge(clk) then
case state is
when write1 =>
data_write<=temp; -- std_logic_vector(counter);
addr<=X"00DFF180";
busstate<="11"; -- Write data
state<=write2;
nWR<='0';
nUDS<='0';
nLDS<='0';
when write2 =>
if clkena_in='1' then
state<=write3;
end if;
when write3 =>
addr<=X"00BFE201";
data_write<=X"0003"; -- Set OVL and LED as output
nWR<='0';
nUDS<='1';
nLDS<='0'; -- Byte write to odd address.
state<=write4;
when write4 =>
if clkena_in='1' then
state<=write5;
end if;
when write5 =>
addr<=X"00BFE001";
data_write<=X"FFFF";
data_write(1)<=temp(6); -- Echo mouse button status to keyboard LED.
nWR<='0';
nUDS<='1';
nLDS<='0'; -- Byte write to odd address.
state<=write6;
when write6 =>
if clkena_in='1' then
state<=read1;
end if;
when read1 =>
addr<=X"00BFE001";
busstate<="10"; -- Read data
state<=read2;
nWR<='1';
nUDS<='1'; -- Byte read from odd address.
nLDS<='0';
when read2 =>
temp<=data_in;
if clkena_in='1' then
state<=write1;
end if;
end case;
end if;
end process;
end architecture;
This state machine reads the value of CIA-A PRA and writes the value read to the COLOR0 register, so the colour of the screen changes in response to the mouse or joystick buttons. It also writes the status of the left mouse button to the LED bit, so the keyboard LED lights in response to the mouse button.
Next I shall test reads and writes to Chip RAM and reads from the Kickstart ROM for reliability.